What is interrupts ?
Interrupt is a signal emitted by hardware or software when a process or an event needs immediate attention.
Interrupt is a signal emitted by hardware or software when a process or an event needs immediate attention. It alerts the processor to a high priority process requiring interruption of the current working process. In I/O devices one of the bus control lines is dedicated for this purpose and is called the Interrupt Service Routine (ISR).
Consider a case, while a car is running, Micro-controller is busy sensing speed of the car, checking other sensors, controlling air conditioner temperature etc. But suddenly an accident happened ! At that time controller stops all the works and go to airbag activation section. So what makes a sudden opening of air bag in seconds? An interrupt signal is used here which has the highest priority of all.
There are software and Hardware interrupts :
- Hardware Interrupts - These occur in response to an external event, like a pin going high or low.
- Software Interrupts - These occur in response to a software instruction.Hardware Interrupts - These occur in response to an external event, like a pin going high or low.
How Does It Work?
When the event or interrupt happens, the processor takes immediate notice, saves its execution state, runs a small chunk of code (often called the interrupt handler or interrupt service routine), and then returns back to whatever it was doing before.
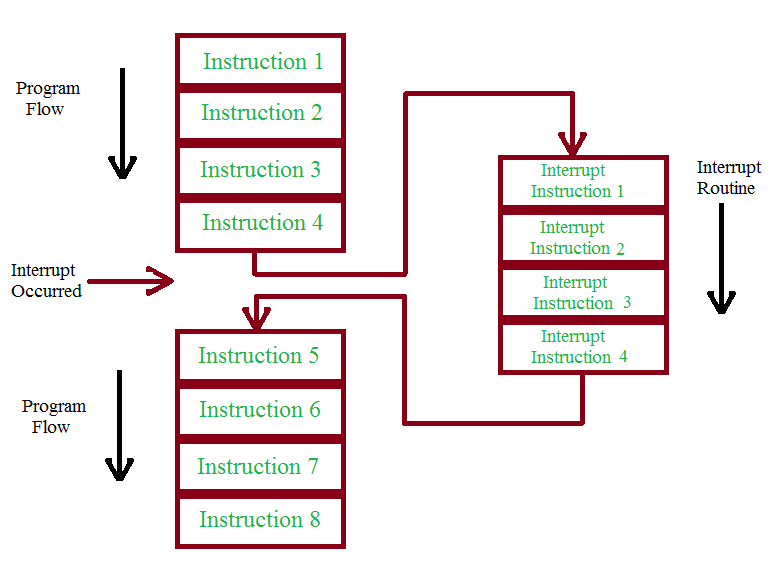
Let's try one simple interrupt using Arduino UNO.
In Arduino function called attachInterrupt() to do this task, its syntax looks like
attachInterrupt(digitalPinToInterrupt(pin), ISR, mode)
- digitalPinToInterrupt(pin):- Pin number of the interrupt, which tells the microprocessor which pin to monitor. The pin depends on the microcontroller being used.
- ISR :- The location of code we want to execute if this interrupt is triggered.
- mode :- defines when the interrupt should be triggered. Four constants are predefined as valid values:
* LOW to trigger the interrupt whenever the pin is low,
* CHANGE to trigger the interrupt whenever the pin changes value
* RISING to trigger when the pin goes from low to high,
* FALLING for when the pin goes from high to low.
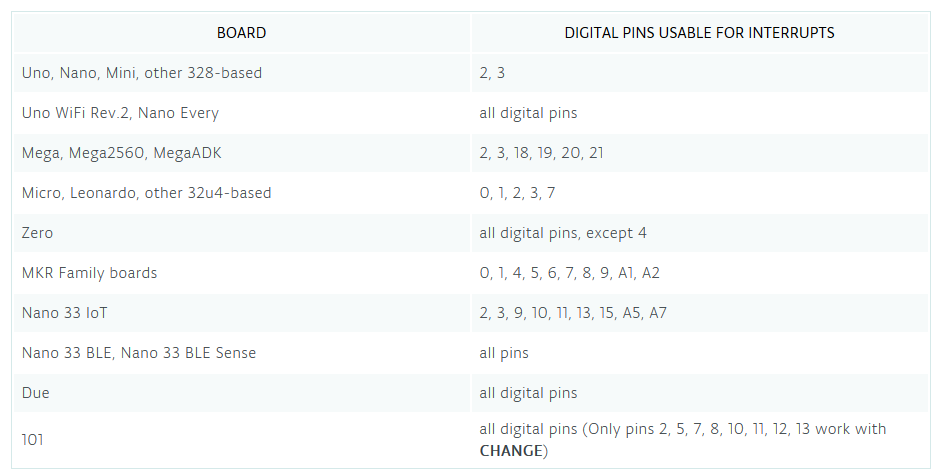
Basic LED ON circuit using interrupt signal
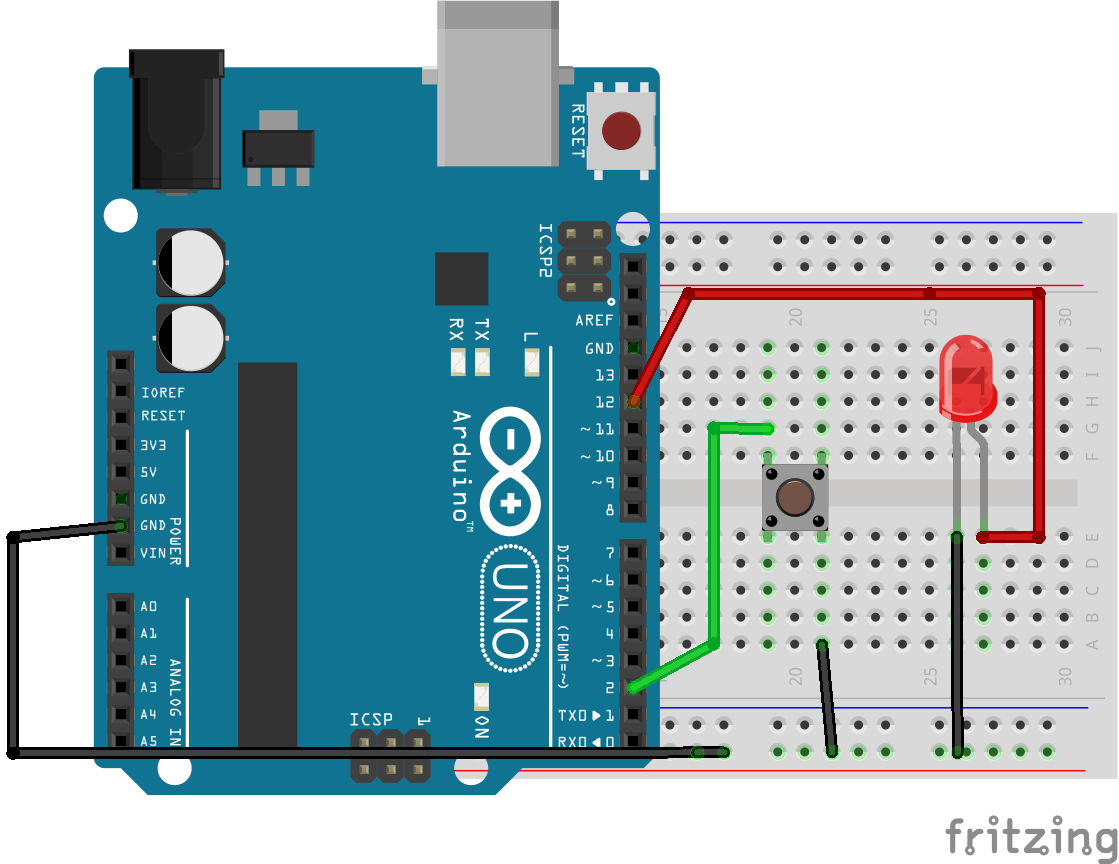
Code !
const int ledPin = 12; //ledPin const int buttonPin = 2; //Button to perform interrupt int x=0, y=0; //some variables to increment int ledToggle = LOW; //led state //variables to keep track of the timing of recent interrupts unsigned long button_time = 0; unsigned long last_button_time = 0; void setup() { pinMode(ledPin, OUTPUT); pinMode(buttonPin, INPUT_PULLUP); Serial.begin(9600); attachInterrupt(digitalPinToInterrupt(buttonPin), button_ISR, CHANGE); } void loop(){ Serial.print("loop " ); Serial.print(x++ ); Serial.println(); delay(500); } void button_ISR(){ button_time = millis(); //check to see if increment() was called in the last 250 milliseconds if (button_time - last_button_time > 250){ Serial.print("Interrupt "); Serial.print(y++); Serial.println(); ledToggle = !ledToggle; digitalWrite(ledPin, ledToggle); last_button_time = button_time; } }
Why that millis() used ?
One common problem with interrupts is they often can trigger multiple times for a single event. if you press the button just once, y will increment many times. A button push might seem like a single step, but in reality the mechanical parts within that button come into contact multiple times before settling into a particular state. There are several ways to remedy this. Often you can fix bounce issues with hardware by adding an appropriate RC filter to smooth the transition. Another option is to address it in software by temporarily ignoring further interrupts for a small time frame after the first interrupt is triggered. that's why millis() is used here.
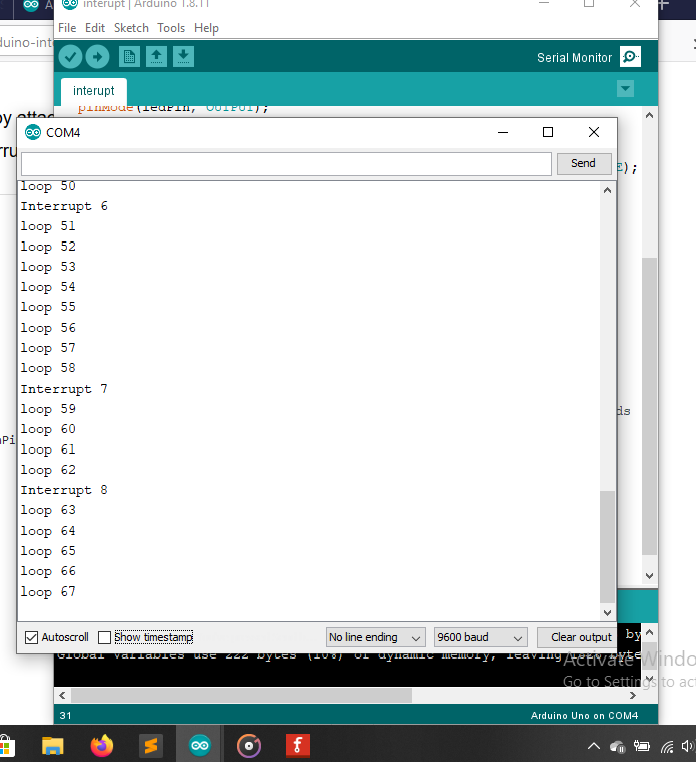
Here controller immediately shifting from loop to interrupt service routine.
This is how an Interrupt can be useful to trigger any important task in between of normal execution.
Interrupt Priority Levels
What happens when two interrupts occur at the same time? Most AVRs do not support what we call interrupt priority levels. Should two interrupts occur simultaneously or there are two or more interrupts waiting in a queue, the priority is determined by the order of their vector addresses. Lower vector addresses are serviced first, Reset will take precedence over all interrupt requests. Again, your datasheet will have more information on your specific board.
Source :-
https://www.arduino.cc/reference/en/language/functions/external-interrupts/attachinterrupt/
https://learn.sparkfun.com/tutorials/processor-interrupts-with-arduino/all